Apr 09, 2020 To authenticate a service account and authorize it to access Firebase services, you must generate a private key file in JSON format. To generate a private key file for your service account: In the Firebase console, open Settings Service Accounts. Click Generate New Private Key, then confirm by clicking Generate Key. What is a Firebase Server Key? A Firebase Server Key and Firebase Sender ID are required in order to send push notifications to Android mobile app devices. The goal of this section is to provision your Firebase Server Key and Firebase Sender ID for use in OneSignal. Apr 10, 2020 To use the Firebase Admin SDK on your own server (or any other Node.js environment), use a service account. Go to IAM & admin Service accounts in the Cloud Platform Console. Generate a new private key and save the JSON file. Then use the file to initialize the SDK.
CONNECTORS TO OTHER SERVICES > Firebase > Tutorials > Authenticate with a Service Account
|
The Transport Tracker is a set of applications that tracks a rangeof moving assets (typically vehicles, such as buses or delivery trucks) andvisualizes them on a map. The solution is based on the shuttle bus tracker atGoogle I/O 2016 and 2017. The code is open sourced on GitHub.You can download it and customize it to suit your needs.
Hint: Try the codelabs for the Transport Tracker backend and map. The codelabs teach you the details of the Transport Tracker backend and of the main frontend display (the map and related HTML components).Introduction
This solution implements asset tracking. For use in a production environment, you therefore need a Google Maps Platform Premium Plan license. For more information, see the Google Maps Platform Terms of Service.
The Transport Tracker consists of the following components:
Data store | A Firebase Realtime Database that stores the vehicle locations, snapped to the road with the Roads API. Firebase provides realtime data synchronization to the backend and map. |
Vehicle locator | An Android app that uses the fused location provider, in the Google Play services location APIs, to report its location to the Firebase Realtime Database. |
Backend | The backend built in Node.js, that processes locations from the Firebase Realtime Database and predicts travel times using the Directions API. |
Map | A web application that uses the Maps JavaScript API to display a styled map showing the bus locations and routes. |
Administrator's overview | A web interface for administrators, giving an overview of the assets being tracked. It displays a map using the Maps Static API, with vehicle and location data from the Firebase Realtime Database. |
Step 1. Get the code
Clone or download theTransport Tracker repo on GitHub.
Step 2. Set up a Firebase Realtime Database

The Transport Tracker uses a Firebase Realtime Database to communicate location updates between the various components of the server and front end applications. When the vehicle locator, or the simulator, stores the location updates in the Firebase Realtime Database, Firebase sends automatic updates to the backend, which in turn updates the front end display.
Set up your Firebase Realtime Database project:
- Go to the Firebase console and click Add project to create a project for your Transport Tracker.
- Enter a project name.
- Click Create Project.
Step 3. Change the Firebase Realtime Database default rules
The Firebase Realtime Database default rules setting should be changed to allow read access.
Change the Firebase Realtime Database default rules setting:
- Go to the Firebase console and open your project.
- Click Database in the left menu and then select the Rules tab.
- Set the default rules setting for
read
totrue
:
Step 4. Get a Google Maps API key
Click the button below, which guides you through the process of registering a project in the Google Cloud Platform Console, activates the required Google Maps Platform and related services automatically, and generates a generic, unrestricted API key.
Make a note of the value of your API key.
During development and testing, you can use a single, unrestricted Google API key for all the Google Maps Platform in your Transport Tracker. When you are ready to move your system into production, you must create separate API keys for each type of API, so that you can secure the keys by HTTP referrer or IP address. For help, see the pre-launch checklist.
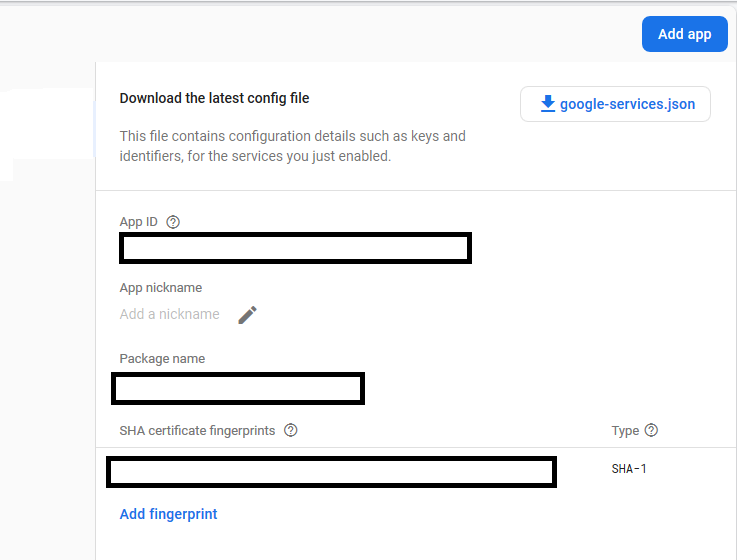
Step 5. Set up the backend
The Transport Tracker backend is an npmNode.js application. It receives realtime updates of the vehicle locations fromthe Firebase Realtime Database, and sets up the panel configurations for use by thefrontend displays. It also includes a vehicle location simulator, so you can runthe Transport Tracker without having to move Android devices aroundto represent your vehicles.
Firebase Generate New Private Key List
Follow these steps to set up the backend:
Get your Firebase web app credentials:
- Go to the Firebase console and open your Firebase project.
- Click Add Firebase to your web app.
- Go to the Service Accounts tab in your Firebase project's settings.
- Click Generate New Private Key and confirm the action as prompted.
- Firebase downloads an
adminsdk
JSON file containing your service account credentials. Store this file for later use.
Optional: You can use Google Cloud services and the Google Cloud Shell to build and run your application:
- Go to Google Cloud Platform console, sign in using your Google Account (Gmail or Google Apps) and create a new project.
- Enter a name for your project, and take note of your allocated project ID.
- Activate Google Cloud Shell from the Cloud platform console, by clicking the button at top right of the Cloud Shell toolbar.
- The Cloud Shell window opens at the bottom of the Cloud console. This is where you can enter commands to interact with the shell. Refer to the Cloud Shell documentation for details.
In Google Cloud Shell, or locally if you're not using Google Cloud, create a
transport-tracker
directory for your application, and copy across thebackend
directory from the cloned GitHub repo:Edit the file
serviceAccountKey.json
, and paste in your Firebaseadminsdk
service account credentials from the file you downloaded earlier. Hint: If you're using the Google Cloud Shell, you can use Cloud's code editor.Edit the
tracker_configuration.json
file and add the following values:mapsApiKey
- your Maps API key. If you don't have one yet, follow the guide to getting an API key.databaseURL
- the address of your Firebase Realtime Database. You can find the URL in the Admin SDK configuration snippet on the Firebase Service Accounts tab.simulation
- a configuration setting that determines whether to use the vehicle simulator or real location data from the vehicle locator. While developing and testing, set this value totrue
.
Run
npm install
to install your dependencies. This may take a few minutes.Run the application:
Open your Firebase Realtime Database to see the results:
- Go to the Firebase console.
- Click the Database tab.
Step 6. Create the map
The Transport Tracker's primary display is a web applicationshowing a styled map from the Maps JavaScript API with markersindicating the locations of the tracked vehicles, retrieved from theFirebase Realtime Database. Panels on the map show the routes and departure times.
Follow these steps to set up the map:
Create a
transport-tracker-map
directory for your application on your server, and copy across all the files from themap
directory in the cloned repo:- Edit the
index.html
file, find the<script>
element at the bottom of the file, and replaceYOUR_API_KEY
with your Google Maps API key. If you don't have an API key yet, follow the guide to getting an API key. - Go to the Firebase console and open your project, then click Add Firebase to your web app. Copy the
config
object that appears on the Firebase console. Theconfig
object contains the Firebase authentication values that you need in the next step. - Edit the
js/index.js
file and populate thefirebaseConfig
object with the Firebase authentication fields and values from theconfig
object that you copied in the previous step. See the Firebase documentation for more information. - Run
npm install
to install your dependencies. Run the application:

Step 7. Set up the vehicle locator Android app
The vehicle locator is an Android app that sends location updates to the Firebase Realtime Database.
Follow these steps to build and run the Android app:
Create a new Android development project:
- In Android Studio, choose File > New > Import project.
- Go to your cloned repo and open the
android
directory in Android Studio.
Connect your app to Firebase using the Firebase Assistant:
- In Android Studio, click Tools > Firebase to open the Assistant window.
- Click to expand one of the listed features (for example, Analytics), then click the provided tutorial link (for example, Log an Analytics event).
- Click Connect to Firebase.
- Go back to Android Studio and enter your project details in the Connect to Firebase dialog that opens there.
- Select Choose an existing Firebase or Google project, then select your Transport Tracker project.
- Click Connect to Firebase, and watch the messages in the Android Studio status bar.
- When the process is complete, a message appears in the Android Studio event log: 'Firebase project created and connected locally to module: app.' Notice the new
google-services.json
file in your project'sapp
directory. - For more information, see the documentation on the Firebase Assistant.
Alternatively, you can connect your app to Firebase manually:
- Go to the Firebase console and open the project you created above.
- Click Add Firebase to your Android app, and follow the prompts.
- Download and save the
google-services.json
file as directed. - For more information, read the Firebase documentation.
Build and run your app. You should see the Transport Tracker startup screen on your Android device, as shown in the screenshot on this page.
Add one or more users to Firebase, so that you can authenticate them to your app:
- Go to the Firebase console and open the project you created above.
Enable email and password as an authentication method:
- Click Authentication, then Set up sign-in method.
- Click Email/Password, then Enable and Save.
Click Add user and specify a valid email address and a password. Take note of the password, because the user needs it to authenticate to your vehicle locator app.
To start tracking on the Android device, enter the following information on the vehicle locator screen:
- Transport ID: A recognizable short identification code for the vehicle you're tracking. This ID appears on the administrator's overview map to indicate the location of the vehicle. For example, use the license plate number of each vehicle as the transport ID.
- Email: The email address corresponding to a user created in the Firebase user management console.
- Password: The password recorded in Firebase for the above user.
Tap Start Tracking. You should see an update in the notification area on your Android device indicating that the Transport Tracker is tracking the device.
Step 8. Set up the administrator's overview
The administrator's overview is a web interface for administrators, showing a summaryof the assets being tracked. It displays a map using theMaps Static API, with vehicle and location data from theFirebase Realtime Database.
Follow these steps to set up the administrator's overview:
Create a
transport-tracker-admin
directory for your application on your server, and copy across all the files from theadmin
directory in the cloned repo:Get your Firebase authentication details:
- Go to the Firebase console and open your project.
- Click Add Firebase to your web app.
Edit the
main.js
file:- Paste your Maps API key into the value for
mapsApiKey
. If you don't have an API key yet, follow the guide to getting an API key. - Paste your Firebase API key into
firebaseApiKey
and the URL of your Firebase Realtime Database intofirebaseDatabaseURL
.
- Paste your Maps API key into the value for
Next steps
Firebase Generate New Private Key West
When you're ready to deploy your system to a production environment, take a lookat the pre-launch checklist.